Event Messaging on the client side with jQuery
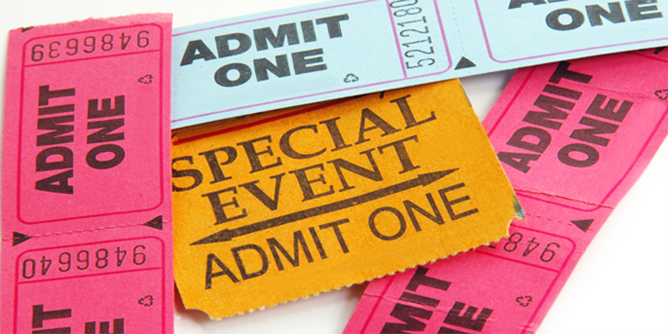
How many times have you come across the following kind of code in JavaScript
// code in script-1.js
// eek, two global variables!
var dialog,
updateMessage = function updateMessage (message) {
dialog.text(message);
}
// function to run when the DOM has loaded
$(function () {
// #dialog is just a simple <p> element
dialog = $('#dialog');
});
then in another script file
// code in script-2.js
// function to run when the DOM has loaded
$(function () {
$('select').change(function () {
// update the dialog with the new selection
updateMessage('the new value is ' + this.value);
});
});
All we’re doing here is updating the text of a <p> element in the DOM whenever the value of a <select> element changes. It’s a contrived and trivial example but it perfectly demonstrates the tight coupling between these two script files; if the updateMessage
function is renamed, removed or modified to reorder the parameters (let’s put aside the use of arguments for a moment), this is going to break script-2 at runtime. What’s more, usually with these kinds of changes, it’s too easy to forget to search through all script and markup-related files for function usages and modify accordingly. And further still, it's polluted the global namespace with two additional variables (a big no no in JavaScript development), dialog
and updateMessage
, to allow script-2 access to the updateMessage function (which needs access to the dialog
variable). There has to be a better way, and there is.
Event Internals in jQuery - Part Two
Part one of jQuery Event internals looked at the DOM Event specifications, the history of the Document Object Model (DOM) event models and reasoning behind why jQuery has an event system. In the second part of this series, we'll be looking at the jQuery.event
object and jQuery.Event
constructor function, both of which play a pivotal role in managing events. Previously, we looked at the bind()
method (and the related specific event handler binding methods such as click()
, keyup()
, etc) and saw that bind()
eventually calls jQuery.event.add
, so let's start by looking at that.
Event Internals in jQuery - Part One
I've been using jQuery for a number of years now and think it's an awesome JavaScript library for providing a rich client experience to web applications with little effort. Whilst I advocate the use of jQuery to quickly and effectively build your client side solutions, I also think that it's important to understand what's going on under the hood, particularly as it can help to quickly pinpoint a bug in your own code (and on the rarest of occasions, a bug in the library code).
ASP.NET MVC 3 IMetadataAware and custom ModelMetadata attributes
UPDATE: I've added a simple example project up on bitbucket to demonstrate the ColorPicker attribute
ASP.NET MVC 3 introduces a new interface, IMetadataAware, for providing additional values to the model metadata at creation time:
/* ****************************************************************************
*
* Copyright (c) Microsoft Corporation. All rights reserved.
*
* This software is subject to the Microsoft Public License (Ms-PL).
* A copy of the license can be found in the license.htm file included
* in this distribution.
*
* You must not remove this notice, or any other, from this software.
*
* ***************************************************************************/
namespace System.Web.Mvc {
// This interface is implemented by attributes which wish to contribute to the
// ModelMetadata creation process without needing to write a custom metadata
// provider. It is consumed by AssociatedMetadataProvider, so this behavior is
// automatically inherited by all classes which derive from it (notably, the
// DataAnnotationsModelMetadataProvider).
public interface IMetadataAware {
void OnMetadataCreated(ModelMetadata metadata);
}
}
Wrap child elements in groups in jQuery
A while ago, I answered a Stack Overflow question about how to wrap child elements matching some selector into groups of a specified size. I had a need for this again recently so I went back to the code, tidied it up and have come up with a slightly more refined plugin.