Event Messaging on the client side with jQuery
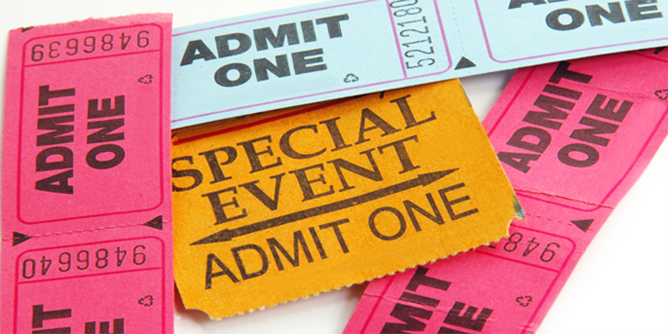
How many times have you come across the following kind of code in JavaScript
// code in script-1.js
// eek, two global variables!
var dialog,
updateMessage = function updateMessage (message) {
dialog.text(message);
}
// function to run when the DOM has loaded
$(function () {
// #dialog is just a simple <p> element
dialog = $('#dialog');
});
then in another script file
// code in script-2.js
// function to run when the DOM has loaded
$(function () {
$('select').change(function () {
// update the dialog with the new selection
updateMessage('the new value is ' + this.value);
});
});
All we’re doing here is updating the text of a <p> element in the DOM whenever the value of a <select> element changes. It’s a contrived and trivial example but it perfectly demonstrates the tight coupling between these two script files; if the updateMessage
function is renamed, removed or modified to reorder the parameters (let’s put aside the use of arguments for a moment), this is going to break script-2 at runtime. What’s more, usually with these kinds of changes, it’s too easy to forget to search through all script and markup-related files for function usages and modify accordingly. And further still, it's polluted the global namespace with two additional variables (a big no no in JavaScript development), dialog
and updateMessage
, to allow script-2 access to the updateMessage function (which needs access to the dialog
variable). There has to be a better way, and there is.